Hello everyone, 👋
How to remove HTML entities from REST API title in WordPress
WordPress supports HTML in fields that developers wouldn’t typically expect HTML in (e.g. post title). When you use the REST API to retrieve posts, the response body will include HTML, including HTML entities. This can be a problem if you are using the REST API to serve data to a non-HTML client, such as a mobile app or a JavaScript library.
To remove HTML entities from the REST API title, you can use the following code:
|
|
To use this code, simply copy and paste it into your theme’s functions.php file. Then, create a new instance of the Customise_WordPress
class. For example:
|
|
This will remove HTML entities from the REST API title for all posts.
You can also modify the code to only remove HTML entities from the REST API title for specific post types. For example, to only remove HTML entities from the REST API title for posts of the post
type, you would change the following line:
|
|
to:
|
|
You can also add additional filters to the add_filter()
call to remove HTML entities from other fields in the REST API response. For example, to remove HTML entities from the REST API content, you would add the following filter:
|
|
The decode_rest_api_content()
function would be similar to the decode_rest_api_title()
function, but it would decode the content
field instead of the title
field.
Benefits of removing HTML entities from the REST API title
There are several benefits to removing HTML entities from the REST API title:
- It makes the REST API response more consistent and easier to parse.
- It reduces the risk of XSS attacks.
- It improves the performance of the REST API, as HTML entities need to be decoded before they can be used.
If you are using the REST API to serve data to a non-HTML client, I recommend that you remove HTML entities from the REST API title and other fields in the REST API response.
Thanks For Reading 🙏
This articles is generated Manually from generative AI, But carefully reviewed by Me personally. Please let me know if you found any issues, in comment section below.
If it is helpful and saves your valuable Time ⏱ please show your support 👇.
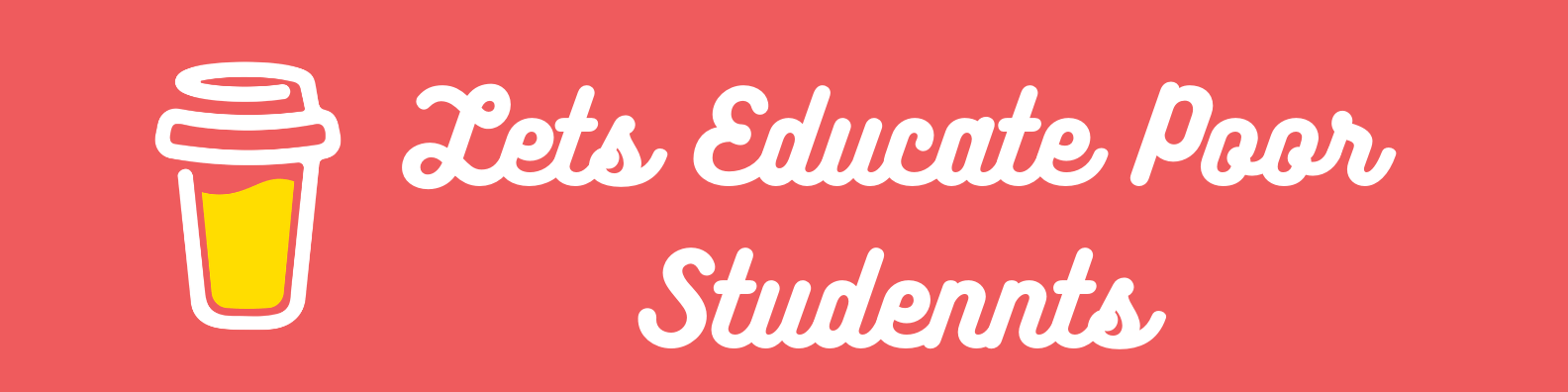
Buy me A Coffee, Thank you and canva ( For Beautiful designs ). Thanks for the reading 👍.